- 目的
- 【novelAI】【python】pythonでnovelAIのプロンプトの内容をpythonで作る(更新)
- 以前作ったものの更新版
- 以前作ったもの
- 前回作ったものとの差分
- 複数の要素を持ったものはランダム関数でランダムな値を取得できるようにした
-
# ピンク - pink hair # 赤 - red hair
- とあったらどちらかが選ばられる
-
- とりあえず入れるやつ(required)を後ろにつけるようにした
- やらないと、品質アップの処理が入らない
- 前に入れるとうまく効かない
- 複数の要素を持ったものはランダム関数でランダムな値を取得できるようにした
- 結論
- 以下のソースを同じディレクトリに配置して実行することでプロンプトの内容が出力される
- 出力結果
- 以下のソースを実行すると得られるプロンプト
-
202402101120 除外したいもの crayon,pen,worst quality,low quality,normal quality,worst quality swept bangs,deformed face,simple background,displeasing very displeasing,longbody,lowres,bad anatomy,bad hands,missing fingers,pubic hair,extra digit,fewer digits,cropped,bad hands,owres,bad anatomy,bad hands,text,error,missing fingers,extra digit,fewer digits,cropped,jpeg artifacts,signature,watermark,username,blurry,missing fingers,bad hands,missing arms,large breasts,ugly face,Outdated Styles,poorly drawn digit,poorly drawn hands 入力コンテンツ seed: ((solo girl)),{school gate},{face close up},{lying},{blush stickers},{tongue out},{green hair},{swept bangs},{middle hair},{ponytail},{no hair accessories},{beautiful detailed eyes},{star-like gold eyes},{red necklace},{slightly bigger breasts},{complicated clothes},{chinese cloths},{wedge heel pumps},{{{{{{masterpiece}}}}}},{{{{{{best quality}}}}}},{{{{{{ultra-detailed}}}}}},{{{{{{illustration}}}}}},{{{{{{detailed cute anime face}}}}}},{{{{{{gorgeous detail}}}}}},{{{{{{extremely detailed}}}}}},{{{{{{very aesthetic}}}}}},{{{{{{cinematic composition}}}}}},{{{{{{no line art}}}}}}
- python
-
#!/usr/bin/python # -*- coding: utf-8 -*- import yaml # ランダム関数を利用するため import random from datetime import datetime # configファイルを読み込ませる YML_FILE = "./config.yml" # 出力ファイルを定義する OUT_PUT_LILE = "./output.txt" # 配列を宣言 # 除外するもの undesired_content = [] # 設定するもの set_content = [] # とりあえず入れるコンテンツ required_content = [] # 実行日付を取得(yyyymmddhhmm) current_datetime = datetime.now() # フォーマットを指定して日付を文字列に変換 date_format = current_datetime.strftime('%Y%m%d%H%M') # 出力例 # 202402050007 # ymlファイルを読み込む with open(YML_FILE, 'r') as file: read_config = yaml.safe_load(file) for content, value_list in read_config.items(): # ループの内容が除外したい項目の場合 if content == "undesired_content": # 除外したい内容の項目でループさせる for value in value_list: # novelAIに書き込む形に変換する undesired_content.append(value) # ファイルに出力する # ファイルを書き込みモードでオープン # wで上書きさせる with open(OUT_PUT_LILE, 'a') as file: # ファイルにテキストを書き込む # 実行日時を出力 file.write(date_format + '\n') # ヘッダー file.write('除外したいもの\n') # 書き込む内容を作成 custom_output_data = ','.join(undesired_content) + '\n' # 内容を書き込み file.write(custom_output_data) # 改行 file.write('\n') # ループの内容がとりあえず入れるやつなら if content == "required": required_content = '{{{{{{' + '}}}}}},{{{{{{'.join(value_list) + '}}}}}}' # ループの内容がコンテンツの場合 # ファイルへの出力を開始しておく with open(OUT_PUT_LILE, 'a') as file: if content == "make_content": # ヘッダー file.write('入力コンテンツ\n') file.write('seed: \n') # 概要の中身でループ for value_content in value_list: # 上記の内容を変数に入れる # all_contentには概要含めて1人分の生成内容が全部入ってる all_content = value_content # all_contentの例 # {'one girl': [{'face': 'baby face'}, {'eye': ['red', 'like a star']}]} for overview in all_content: # overviewの例 # solo girl # とりあえず入れるやつを出力 # これは一番最後に入れる # file.write(required_content + ',') # 概要を出力 file.write('((' + overview + ')),') # one_contentには1人分の生成内容が全部入ってる one_content = all_content[overview] # one_contentの例 # [{'face': 'baby face'}, {'eye': ['red', 'like a star']}] for element in one_content: # element = 要素 # elementの例 # {'background': ['sandy beach', 'snowing', 'forest']} # {'body': ['face close up']} # {'pose': ['sexy pose']} for key, value in element.items(): # keyにはパーツの名称 # 例: # face # eye # valueにはパーツの内容が入ってる # 例: # baby face # ['red', 'like a star'] # 要素数を変数に入れる value_len = len(value) # valueがないものは次に進む if value_len == 0: continue # value_lenの値が1の場合 if value_len == 1: # 内容を書き込む file.write('{' + ' },{ '.join(value) + '}') # value_lenの値が複数の場合 else: # valueの値を要素数で取得するため-1する # 配列の要素は0から取得するため value_len_fix = value_len - 1 # value_lenの中からランダムな値を取得する value_random = random.randint(0, value_len_fix) # 書き込む内容を変数に入れる write_value = value[value_random] # 内容を書き込む file.write('{' + write_value + '}') # typeによって条件分岐する処理はコメントアウト # 用意したymlでstrのものはない # valueが'list'か'str'かで処理を分ける # valueのtypeを確認 # value_type = type(value) # # typeが'str'の場合 # if value_type == str: # # 内容を書き込む # file.write('{' + value + '}') # # typeが'list'の場合 # if value_type == list: # # 内容を書き込む # file.write('{' + ' },{ '.join(value) + '}') # keyごとに','で区切る file.write(',') file.write(required_content) # one_contentの区切りとして最後に'}'を記載する file.write('\n\n')
-
- ymlファイルの中身
-
# 除外したいものリスト undesired_content: # クレヨン - crayon # ペン - pen # 低いクオリティ - worst quality - low quality - normal quality # 品質の悪い前髪 - worst quality swept bangs # 変形した顔 - deformed face # シンプルな背景 - simple background # 非常に不快な - displeasing very displeasing - longbody - lowres - bad anatomy - bad hands - missing fingers - pubic hair - extra digit - fewer digits - cropped - bad hands - owres - bad anatomy - bad hands - text - error - missing fingers - extra digit - fewer digits - cropped - jpeg artifacts - signature - watermark - username - blurry - missing fingers - bad hands - missing arms - large breasts # 容姿が醜い顔 - ugly face # 時代遅れの - Outdated Styles # 指の書き方が下手 - poorly drawn digit # 手の書き方が下手 - poorly drawn hands # とりあえずいれるやつ required: - masterpiece # 最高品質 - best quality - ultra-detailed - illustration # 詳細なかわいいアニメの顔 - detailed cute anime face # ゴージャスなディテール - gorgeous detail # 非常に詳細な - extremely detailed # 非常に美しい - very aesthetic # 映画のような構成 - cinematic composition # 線画なし - no line art # 出力する内容 make_content: # 女の子の例2 - solo girl: # 背景 - background: # 砂浜 - sandy beach # 雪 - snowing # 森 - forest # 洋風の部屋 - Western-style room # 校門 - school gate # 教室 - classroom # 教室の窓際 - by the classroom window # 花畑 - flower garden # 舞っている花びら - falling petals # 雪景色 - winter, snow # 平原 - plains # 水中 - under water # 道路 - road # 海辺 - seaside # プールサイド - Poolside # 水の反射 - reflection water surface # 秘境 - fairyland # どこを生成する? - body: # 全身 #- whole body # 顔アップ - face close up # ポーズ - pose: # セクシー - sexy pose # 座ってる - sitting # 腕組み - crossed arms # 膝を抱えて座る - sit with one's knees # 膝を抱えてベンチに座る - sit on a bench holding your knees # 振り返ってる - looking back # 横たわってる - lying # 足を組む - cross-legged # 前屈みにする - leaning forward # ものを抱きしめる - object hug # 腰に手を当てる - hand on hip # 顔の特徴 - face: #- baby face # ロリ # - loli # 笑顔 - smile # 喜び - happy # ウィンク - one eye closed # 恥ずかしそうな - blush # 喜びつつ恥ずかしそう - blush stickers # 顔全体が恥ずかしい - full-face blush # 口 - mouth: # 舌出し - tongue out # 口を開ける - open mouth # 口を閉じる - close mouth # 尖った歯 - sharp teeth # 髪色 - hair_color: # ピンク - pink hair # 赤 - red hair # 青 - blue hair # 金 - gold hair # 黄 - yellow hair # 緑 - green hair # 茶 - brown hair # オレンジ - orange hair # 紫 - purple hair # グレー - gray hair # 前髪 - bangs: # 横に流す - swept bangs # 髪の長さ - hair_length: # 大きい髪 #- big hair # 小さい髪 # - small hair # 中くらい - middle hair # 髪型 - hairstyle: # 髪型 # ポニーテール - ponytail # 低い位置のポニーテール - Lower ponytail # ハーフアップ - Half-up hairstyle # 髪のアクセサリー - hair_accessories: # 赤いティアラ - wear a red tiara on your head # 髪のアクセサリーはなし - no hair accessories # 目でとりあえず入れる - eye_required: # とりあえず入れておく - beautiful detailed eyes - eye_type: # 星のような赤い目 - star-like red eyes # 星のような赤い目 - star-like pink eyes # 星のような赤い目 - star-like blue eyes # 星のような赤い目 - star-like gold eyes # 星のような赤い目 - star-like yellow eyes # 星のような赤い目 - star-like green eyes # 星のような赤い目 - star-like brown eyes # 星のような赤い目 - star-like orange eyes # 星のような赤い目 - star-like purple eyes # 星のような赤い目 - star-like gray eyes # 首 - neck: # 首には赤いネックレス - red necklace # 胸 - breasts: # 大きい胸 - big breasts # 普通の胸 - medium breasts # 少し大きい胸 - slightly bigger breasts # 小さい乳首 - small breasts # ヌード入れないとバグる #- small nipples # 下着 # 入れると下着姿になってしまう # - underwear: # # 赤いブラジャー # - red bra # # 赤いパンティ # - red panties # 服装 - clothing_required: # 複雑な服 - complicated clothes - clothing_type: # 光を入れる #- let in light # 下着 - underwear # スクール水着 - school swimsuit # マイクロビキニ - micro bikini # スポーツブラ - sports bra # ゴスロリ - Gothic and Lolita clothes # 黄色のワンピース - One piece to wear to dance # 和服 - Japanese clothes # 着物 - kimono # 巫女服 - miko clothes # チャイナ服 - chinese cloths # 学制服 - school uniform # ウェディングドレス - wedding dress # ニット - knit # 軍服 - military uniform # メイド服 - maid clothes # アイドル - idol costume # ドレス - dress # パーカー - hoodie # セーター - sweater # ブレザー - blazer # ショートパンツ - shorts # ワンショルダービキニ水着 - one shoulder bikini # ビスチェ水着 - bustier swimsuit # モノキニ水着 - monokini swimsuit # ビキニの水着 - bikini swimwear # 靴 - Shoes: # 靴はウェッジヒールパンプス #- Shoes are wedge heel pumps - wedge heel pumps # 裸足 - barefoot # ブーツ - wearing boots
【novelAI】【python】pythonでnovelAIのプロンプトの内容をpythonで作る(更新)
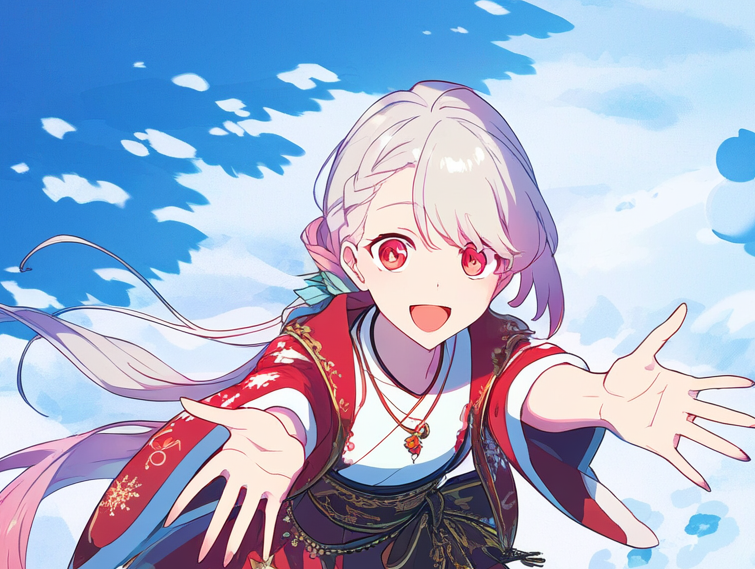